Static code analysis is the process of examining source code without executing it. The goal is to identify potential issues, vulnerabilities, and quality problems quickly. By analyzing code in its "static" state, that is: before compilation or runtime, development teams can catch bugs, security flaws, and maintainability issues that might otherwise remain hidden until later stages when they become more costly to fix, or that might even make it all the way to production, with potentially catastrophic consequences.
The low cost and short feedback loop of static analysis mean that developers can act on the findings immediately, often inside their editors or IDEs. When a developer makes a typo in a variable or method name, their IDE decorates it with a squiggly line.
That’s static code analysis in action. The developer sees their mistake and fixes it in seconds, and they don’t break their flow. Without that rapid feedback, the error would show up much later. Depending on the programming language and the environment, this could mean discovering the mistake minutes, hours or days later, and then going back, reopening the file, finding the line, fixing the mistake and slapping one’s forehead.
The speed and availability of static code analysis throughout the development process has other benefits. Manual code reviews can be an important part of ensuring code quality, but they are time-consuming and sometimes become a bottleneck. Even simple static analysis reduces the load on code reviewers by catching low-level mistakes, enforcing code formatting standards and generally removing other sources of noise so that reviewers can read faster and focus on the important parts.
More advanced static code analysis tools can go much further, identifying patterns or security issues that even expert reviewers might occasionally miss. Static code analysis tools automate this inspection process, providing consistent and thorough evaluations that help teams deliver more reliable, secure, and maintainable software.
Static vs Dynamic Code Analysis
You might ask: why static analysis? Dynamic code analysis sounds more powerful, and in some cases it is.
Code coverage analysis is one of the most common uses of dynamic code analysis: a code coverage tool like Jacoco, Cobertura or Clover instruments your code while your tests run, and checks which paths are executed by the tests. Performance profiling is another example.
There are a number of tradeoffs that are important to understand when thinking about a comprehensive testing strategy. While both approaches aim to improve code quality, they operate at different stages of the development process, identify different types of issues and come with different costs.
Static code analysis
- When it happens: At any time during the development process, including in CI/CD pipelines.
- What it examines: Source code files
- How it works: Parses code and analyzes patterns
- What it finds: Syntax errors, style violations, type errors, potential bugs, security vulnerabilities, complexity issues
- Example tools: SonarQube, CodeScene, ESLint, PMD, Checkmarx
Dynamic code analysis
- When it happens: The code must be running: sometimes on a developer’s workstation but more often inside a testing environment
- What it examines: Application behavior during execution
- How it works: Monitors application as it runs with test inputs, sometimes using instrumentation
- What it finds: Code coverage, memory leaks, performance bottlenecks, actual runtime exceptions, integration issues
- Example tools: Valgrind, JProfiler, Application Performance Management (APM) tools
Aspect |
Static Analysis |
Dynamic Analysis |
Code execution | No execution required | Must run the code |
Coverage | Can analyze entire codebase | Only analyzes executed paths |
False positives | Higher rate of false positives | Fewer false positives |
Timing |
Throughout the development cycle |
Later in the development cycle |
Required environment |
Access to source code |
Complete runtime environment |
Resource usage | Generally less resource-intensive | Can be resource-intensive |
Security testing | Finds potential vulnerabilities | Confirms exploitable vulnerabilities |
Which is the best choice? The most effective quality assurance strategies incorporate both static and dynamic analysis, as they complement each other's strengths and weaknesses. Static analysis catches issues early, while dynamic analysis verifies how code behaves in actual execution environments.
How Does Static Code Analysis Work?
Static code analysis tools can employ a wide variety of techniques to inspect source code without executing it.
1. Lexical analysis
Nearly all non-trivial static code analysis tools begin with this step. The code is broken down into tokens—the syntactical building blocks of the programming language (keywords, identifiers, operators, etc.). This transformation allows the analyzer to work with a more structured representation of the code.
2. Abstract syntax tree (AST) generation
Some tools will then use the tokens from the lexical analysis, combined with knowledge of the programming language, to construct an Abstract Syntax Tree (AST). The AST represents the hierarchical structure of the code, making it easier to analyze relationships between different code elements.
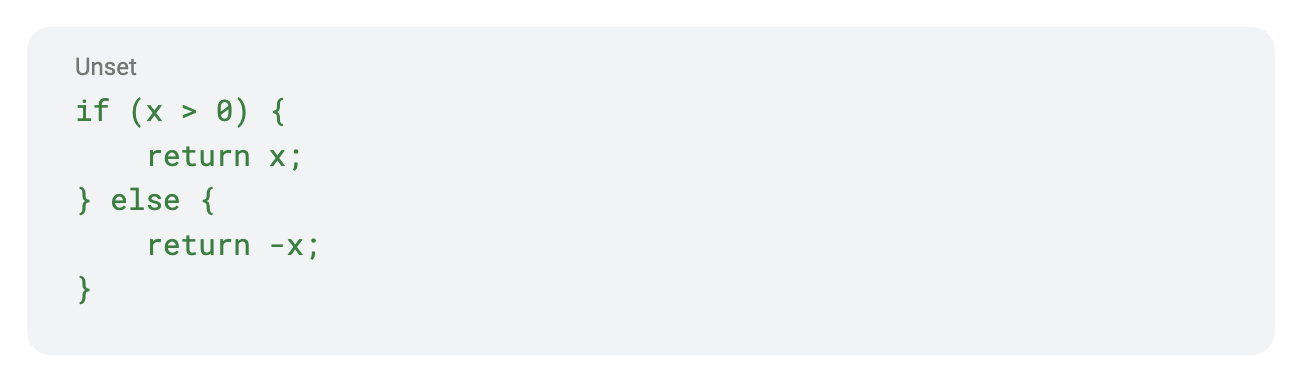
3. Control flow analysis (CFG)
By analyzing how control transfers between statements, it is then possible to build a Control Flow Graph (CFG) that maps all possible execution paths. This enables the detection of unreachable code, infinite loops, and other flow-related issues.
4. Data flow analysis
Data flow analysis tracks how data values move through the program, identifying issues like uninitialized variables, unused variables, and potential null pointer dereferences.
5. Semantic analysis
The analyzer evaluates the meaning of the code, checking for type mismatches, incorrect API usage, and logic errors that might be syntactically correct but semantically problematic.
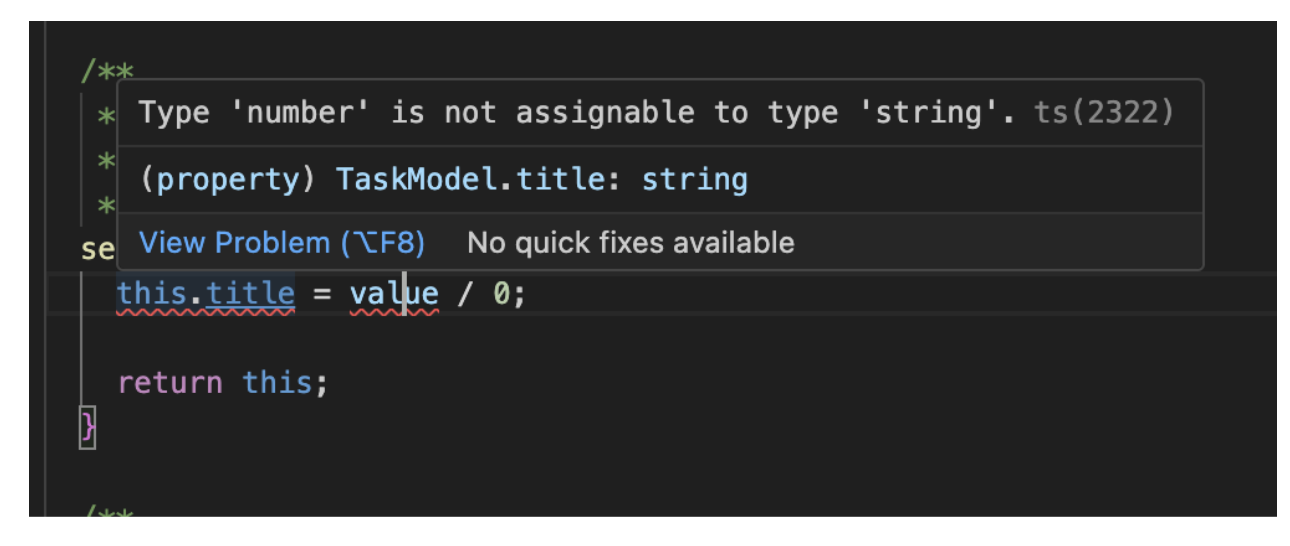
6. Pattern matching
Many static analyzers search for known problematic code patterns or anti-patterns that indicate potential bugs, security vulnerabilities, or design issues.
7. Rule enforcement
Rule enforcement is more an interpretation of the results of the analysis than an analysis technique. We can include it here, though, because the ability to define rules is an important interface between the user and the analytical tool.
Static analysis tools can apply a set of predefined or custom rules that enforce measurable characteristics when changes are made to a codebase. Organizations may choose to be more or less strict with certain aspects of the analysis, or to give more weight to some characteristics than others. These choices are contextual and depend on the technologies involved as well as the priorities of the organization: high performance, high reliability, high development speed, low technical debt. Customizing an analysis is a way to adapt the analytical tool to the circumstances where it is used.
Types of Static Code Analysis
Static analysis can also be classified by the different purposes that it serves. Different tools focus on different aspects of the code they analyze:
Style analysis
Style analysis enforces coding conventions and formatting standards to ensure consistency across a codebase. While these issues don't affect functionality, consistent style improves readability and maintainability, and becomes especially important in large companies. Often these tools can automatically correct indentation and spacing, this relieving developers of this responsibility.
Examples include checking for:
- Proper indentation and spacing
- Naming conventions
- File organization
- Comment requirements
Tools: ESLint, FxCop, RuboCop, Prettier
Structural analysis
Structural analysis examines relationships between code elements, identifying architectural and design issues that could impact maintainability.
This includes detecting:
- High coupling between components
- Circular dependencies
- Architectural constraint violations
- Package structure issues
Tools: Structure101, JDepend, CodeScene
Code quality analysis
Code quality analysis identifies potential bugs, code smells, and maintainability issues that could lead to problems over time.
This involves detecting, for example, things like:
- Unused variables and dead code
- Duplicate code (copy-paste detection)
- Overly complex methods and classes
- Resource leaks
Tools: SonarQube, PMD, CodeScene
Security analysis (SAST)
Static Application Security Testing (SAST) focuses specifically on identifying security vulnerabilities.
This includes finding:
- Input validation issues
- SQL injection vulnerabilities
- Cross-site scripting (XSS) opportunities
- Insecure cryptographic implementations
- Authentication weaknesses
Tools: Checkmarx, Fortify, Snyk, Aikido
Formal methods analysis
This advanced form of static analysis uses mathematical techniques to formally verify that code meets specific correctness properties.
Applications include:
- Critical systems verification
- Protocol implementation verification
- Concurrent code correctness
Formal methods analysis, however, has so far proven to be impractical except in certain niche applications, and generally remains a theoretical pursuit. If you’re unsure whether formal methods analysis is right for you or not, then it’s probably not.
Tools: TLA+, the SPARK programming language, Frama-C
Benefits of Static Code Analysis
Implementing static code analysis provides numerous benefits throughout the development lifecycle:
1. Early feedback
Finding bugs early in the development process significantly reduces the cost of fixing them. Industry research consistently shows that bugs caught in development cost 5-10 times less to fix than those discovered in production. Many static analysis tools are very fast and therefore easy to use at multiple steps during the process. The fastest tools can be integrated into code editors and IDEs, thus reducing the feedback loop to the absolute minimum.
Agile software development, the Devops and Continuous delivery movements, along with proponents of Test-driven development (TDD) have all shown how shortening the feedback cycle improves both quality and productivity. Static code analysis can be a key part of these processes by accelerating the feedback.
2. Improved code quality
By enforcing standards and identifying code smells, static analysis tools help maintain higher overall code quality, leading to more maintainable and reliable systems.
3. Enhanced security
Security-focused static analysis can identify vulnerabilities before they make it into production, reducing the risk of security breaches. This is particularly important for applications handling sensitive data or critical infrastructure.
4. Improved developer skills
Developers learn from static analysis feedback, gradually improving their coding practices and developing awareness of common pitfalls.
5. Consistent code reviews
While not replacing human code reviews, static analysis tools can provide a consistent baseline that ensures no code escapes basic quality checks. This also reduces the load on the human reviewers. When the low-level issues are taken care of automatically, reviewers can focus on more complex and subtle problems, where their expertise is more valuable.
6. Regulatory compliance
Many industries have regulatory requirements for code quality and security. Static analysis can help document compliance with these standards.
7. Accelerated development
Despite the initial overhead, static analysis ultimately speeds development by reducing rework and debugging time.
8. Accelerated deployment
As the software industry has moved from waterfall development release cycles, to CI/CD and now to Continuous deployment, the speed of delivery depends more and more on the tooling. Automated checks performed by static analysis tools play an essential role in the process of reducing cycle times to minutes.
Limitations of Static Code Analysis
While powerful, static code analysis can’t solve all our problems. Being aware of this is important. These kinds of analysis tools offer important information but we can’t pretend that they are infallible.
1. False positives
Static analysis tools often flag issues that aren't actual problems in practice. These false positives can lead to "alert fatigue" if not managed properly. This is one of the main reasons why the choice of a tool can be critical. Careful configuration of the rules can reduce this, but not eliminate it entirely.
2. False negatives
No static analyzer can find all possible issues. There are entire categories of bugs that cannot be caught through static analysis. Static code tools, unlike tests, do not know what your code is trying to accomplish. By automating part of the process, static analysis can reduce some of the bughunting workload.
3. Limited runtime context
Static analysis cannot account for all possible runtime states, making it difficult to identify issues that only manifest under specific execution conditions.
4. Performance overhead
Automation can speed up the review process, as mentioned above. However, all that analysis does come at a cost. Comprehensive static analysis can be computationally expensive, potentially slowing down build processes if not optimized. Judicious use and configuration of the tools is important here as well.
5. Learning curve
Teams must invest time in learning how to interpret and respond to analyzer results, as well as how to configure tools appropriately for their codebase and organizational context.
6. Language limitations
Different analyzers support different languages with varying degrees of sophistication. Analysis quality can vary significantly between programming languages.
How to Pick a Code Analysis Tool
To selecting the right static analysis tool for you, here are some factors to consider:
1. Language support
Ensure the tool fully supports your programming languages and frameworks. Deep language understanding results in more accurate analysis. All tools are not equal for all languages.
2. Integration capabilities
Most modern tools should integrate well with your tooling: your development environment (editor, IDE, Git hooks…) and/or your CI/CD pipeline. Still, it’s important to look at how this integration actually works: are their plugins for the IDEs used by your developers? Does the tool require special resources that would make it hard to run as part of your build pipeline?
3. Customizability
Every situation is different. Getting relevant, meaningful results from a tool often means adapting it to your particular circumstances. Look for tools that allow you to:
- Enable/disable specific rules
- Adjust rule severity levels
- Create custom rules for organization-specific concerns
- Fine-tune rules on a per-directory or per-team basis
- Configure analysis scope
4. False positive management
Dealing with false positives can be an enormous time sink, or lead to simply ignoring results. It’s important to consider how the tool handles false positives and how this will fit into your workflow:
- Can issues be suppressed or marked as false positives?
- Does the tool learn from feedback?
- How granular is the control over rule sensitivity?
5. Reporting and visualization
An analysis that no one looks at or understands won’t result in any improvements being made. Reporting and clear visualizations are essential. It’s important to think about how the information from the static analysis will flow through your organization.
- Are reports clear and actionable?
- Can results be visualized in meaningful ways?
- Are trend analyses available to track improvements?
- Can reports be customized for different stakeholders?
6. Performance
Assess the tool's performance characteristics:
- How long does it take to analyze your codebase?
- Can the analysis be incremental (analyzing only changed files)?
- Are there options for parallel processing?
7. Community and support
A strong community or commercial support ensures:
- Regular updates and rule improvements
- Help when issues arise
- Knowledge sharing around best practices
8. Total cost of ownership
Consider all costs involved:
- License fees (if commercial)
- Infrastructure requirements
- Integration effort
- Maintenance overhead
- Training requirements
Best Practices for Rolling out Static Code Analysis
Here are some best practices to maximize the value of static code analysis:
1. Integrate early in the development process
Run static analysis as early as possible:
- Configure IDE plugins for real-time feedback
- Run analysis on pre-commit hooks
- Integrate into CI/CD pipelines, on pull requests or as quality gates at other steps in the process
2. Start small and expand gradually
Begin with a manageable set of high-value rules:
- Focus initially on critical issues (security, major bugs)
- Gradually introduce style and maintainability rules
- Allow time for teams to adapt to new requirements
If your tool allows it, you might also consider limiting the scope to certain parts of your code base or certain teams. This can be a low-risk way of building up some institutional knowledge that can be spread later. The early adopters can help onboard those who come next, and their initial acceptance of the tool creates a precedent. “If they can do it, maybe I can too!”
3. Customize rules to your context
Tailor analysis to your specific needs:
- Disable rules that don't apply to your codebase
- Adjust severity levels based on your priorities
- Create custom rules for organization-specific patterns
This is another reason to start small, as mentioned in the previous point: early adopters will also provide feedback that can help fine-tune the analysis rules. When you push out the tooling to the rest of the organization, many of the early issues will already be resolved.
4. Establish a clean baseline
For existing codebases:
- Run initial analysis to establish a baseline
- Address critical issues immediately
- Create a plan for gradually addressing technical debt
- Consider using "new code" rules to prevent regression
Legacy projects can be especially challenging because you may be confronted early on with so many things to fix that you don’t know where to start, or feel overwhelmed by the sheer volume. Introducing a prioritization strategy can be decisive in these cases.
5. Manage false positives effectively
Develop a process for handling false positives:
- Use suppressions sparingly and specifically
- Review and document suppressed warnings
- Periodically revisit suppressed issues
When you see patterns in the suppressions, it’s time to be analytical. It’s tempting to think that the problem is the rules themselves. And sometimes that’s true. Still, it’s always a good idea to step back and think about what could be causing repetitive violations of the same rules. Are there company coding practices that are out of sync with industry standards? Is the level of technical debt so high that developers are having a hard time doing the right thing while still meeting deadlines? Before simply adjusting the rules, a root cause analysis can be an eye-opener.
6. Incorporate into code reviews
Use static analysis results in code reviews:
- Require clean static analysis before review
- Focus human review on aspects that tools can't evaluate
- Use tool findings as discussion points for improvement
7. Monitor
Track analysis metrics to measure improvement:
- Overall issue count and density
- Issues by category and severity
- Fix rate and introduction rate
The trend is often more important than the raw values. Improvements can take time, especially on large projects. Ensuring that things are going in the right direction is essential.
8. Provide developer training
Ensure developers understand:
- The purpose and value of static analysis
- How to interpret and address findings
- How to provide feedback on false positives
- How to write code that passes analysis
9. Balance automation and human judgement
Remember that tools complement rather than replace human expertise:
- Use tools to handle routine checks consistently
- Preserve time for creative problem-solving
- Allow overrides with proper justification and documentation
10. Continuously evolve your approach
Regularly review and refine your static analysis strategy:
- Update rule sets as your codebase matures
- Evaluate new tools and techniques
- Collect feedback from development teams
- Measure impact on quality metrics
It’s hard to overstate the importance of continually adapting your static analysis strategy. The greatest danger of automated feedback is that it just becomes “noise” for the developers it is supposed to be helping. Keeping a close eye on the interactions between your teams and the static analysis tools they are using will ensure that they will continue to provide value.
How CodeScene Enhances Static Code Analysis
CodeScene innovates in the static code analysis domain in two different ways. With Code Health, CodeScene introduces a new metric that is based on static analysis that identifies patterns indicative of code smells. In addition, CodeScene’s behavioral analysis uses inputs that are not available to static analysis tools and provides crucial context that helps make better decisions about your projects.
What is Code Health?
Code Health is a static code analysis metric that detects over 25 widely recognized code smells that have demonstrated negative impacts on code maintainability. As such, it is a technique for measuring technical debt in a codebase. How hard is it to make changes to a particular file or set of files? Managing complexity is the greatest challenge for developers when they work with existing code. Code Health is a way of quantifying this aspect of the interaction between code and developers.
Research conducted by CodeScene and academic partners has shown that Code Health is a reliable way of predicting positive or negative outcomes. See the “Code Red” research for more information about this research.
Behavioral Code Analysis
CodeScene goes beyond conventional static analysis by examining how developers actually interact with the code that they work on. To do this, CodeScene brings in other data sources, the most important of which is the entire commit history of your project.
By analyzing commit patterns, change frequencies, and developer collaboration behaviors, CodeScene provides a deeper understanding of your real situation of your project:
- Hotspots: You see, not all code is equally important. As shown in the visualization below, development activity tends to cluster in a small part of the codebase. Hotspots are a behavioral code analysis technique which identifies those critical parts based on data from version-control. In practice, this means that any Code Health issues in a hotspot tend to become major productivity bottlenecks – it’s high-interest technical debt. (See this blog on prioritizing technical debt for more details on hotspots).
Figure 1: Example on Hotspots in a codebase. Visualized via CodeScene.
- Knowledge distribution: When developers leave, the organization loses their detailed knowledge of specific areas of a codebase. This is another form of risk that needs to be managed. Identifying the impacted areas is an important step in mitigating this risk.
- Team coupling: By tracking where team members make changes, CodeScene can see whether your team structure and your architecture are working together, or against each other.
Prioritized findings
One of the most common drawbacks of static analysis tools is their capacity to quickly flag so many problems – often in the thousands – that it seems impossible to act upon them. Behavioral analysis comes to the rescue here. When we know which areas of a project are the most significant in tees of development effort and technical debt, we can carefully target our actions for maximum benefits. As mentioned above, “alert fatigue” and a low signal-to-noise ratio are two factors that can defeat a static analysis strategy. Behavioral prioritization helps bridge the gap between data and action.
Instead of overwhelming teams with thousands of issues, CodeScene helps prioritize remediation efforts by identifying:
- High-risk code with rapid change rates
- Issues in code with high business impact
- Technical debt in frequently modified areas
Temporal perspective
CodeScene's unique temporal analysis reveals:
- How code complexity evolves over time
- Which refactoring efforts have been most effective
- Where technical debt is accumulating fastest
Integration with development workflows
CodeScene seamlessly integrates with:
- Git repositories for historical analysis
- CI/CD pipelines for continuous monitoring
- Pull request workflows for just-in-time feedback
By combining traditional static analysis with behavioral insights, CodeScene provides a more nuanced and actionable understanding of code quality, helping teams make data-driven decisions about where to focus improvement efforts.
Conclusion
Static code analysis represents a crucial component of modern software development practices. By identifying issues early, enforcing standards consistently, and providing objective quality metrics, static analysis tools help development teams build more reliable, secure, and maintainable software.
While no static analysis tool can find all potential problems, and human judgment remains essential, these tools significantly can be an essential part of your software development process, and a key to assuring that your process aligns with your priorities, whether that is development velocity, security, defect reduction or long term reduction of technical debt. The initial investment in setting up and learning to use static analysis tools pays dividends throughout the software lifecycle in reduced debugging time, fewer production incidents, and more maintainable codebases.
As development practices continue to evolve with trends like DevOps, continuous delivery, continuous deployment, and microservices, static analysis tools are adapting to provide faster feedback, better integration with development workflows, and more sophisticated analysis techniques. Organizations that effectively integrate static analysis into their development processes gain a competitive advantage through higher quality, improved security, and more efficient development processes.
With the information and best practices outlined here, you will be able to make informed choices as you explore this area and choose and adopt the available tools to fit your organization’s needs, technical environment and specific circumstances. Static code analysis is certainly not a silver bullet but when used intelligently it can provide a wide range of benefits, both in terms of efficiency and quality.